Out of the new HTML tags one that you should be really excited about is the slider input tag. It used to be that you could only render a slider bar with a javascript library, but not anymore.
What Can This New Tag Do
The slider tag allows you to render a slider whose position represents a value in a range you specify. The most left side of the bar is the minimum value, the right most is the maximum.
Your users can slide the bar to the left or right to choose the value they like.
What Does The Slider Look Like
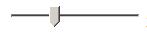
HTML5 Slider In Google Chrome
That was a picture of the bar in my browser but now look at it live
If you see a textbox above this sentence instead of the slider you browser does not support this tag yet.
How To Use It
Since the slider is used for users input it used the same syntax other input tags do. The type of this tag though is "range"
Basic
<input type="range" />
Max and Min
The previous snippt should be enough to show the slider, but this tag has more attributes you can use. Let’s set the range of values (max and min).
<input type="range" min="0" max="100" />
Now users can choose any number between 0 and 100.
Set Default Position
The slider has a default value of zero but if you give it a different value, when the browser renders the code, the pin will be positioned at that value. The next code will position the pin halfway between the ends of the bar.
<input type="range" min="0" max="50" value="25" />
Set Steps
What if you just want people to choose values at intervals of 5? This can easily be accomplished using the step attribute.
<input type="range" min="0" max="50" value="25" step="5" />
Display Values
How will users know what value they are choosing? That’s a good question and the answer is that you are going to have to come up with your own solution.
I wrote a simple javascript code that will show the value of the slider as you slide it.
<html> <body> <input type="range" min="0" max="50" value="0" step="5" onchange="showValue(this.value)" /> <span id="range">0</span> <script type="text/javascript"> function showValue(newValue) { document.getElementById("range").innerHTML=newValue; } </script> </body> </html>
And that should render this (if your browser supports it)
0
How To Use With PHP
Since this is an input tag all you have to do is give it a name attribute inside a form tag and get the value just like you would for a text field.