I just had an idea for a website, it uses the Best Buy’s Remix API so I had to learn to use it and now I want to show you how easy it is.
About The API
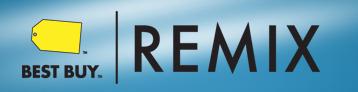
Best Buy's API
You can only do one thing with this API and that is search. But search is subdivided into six sections:
- products
- stores
- categories
- reviews
- search
- and search bias
I will show you how to use the second to last category, this is the same as product search except that it’s queries are far more flexible.
Getting Started
The first thing you will need is an application key. You can get one for free by heading over to this site.
After you have registered yourself and your application you should be greeted with a screen like this one. The Remix API is all you need.
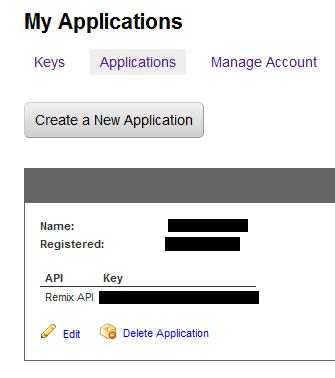
Best Buy Applications Manager
Just in case you can’t find it, the link to this screen is thisĀ http://remix.mashery.com/apps/myapps
Your First Search Query
Each query you make to Best Buy must consist of the keywords you are looking for and your ApiKey, everything else is optional but we will use what I’ve circled below.
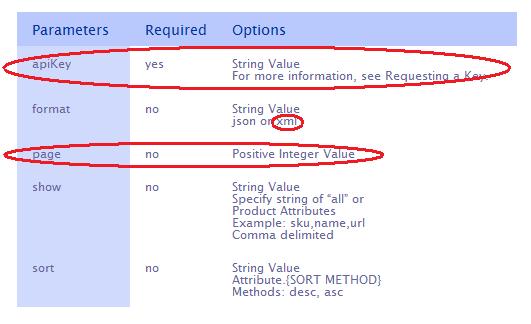
BestBuy API Parameters Table
The default format is XML so we won’t even have to mess with that. If your query exceeds ten results they will be separated in to pages, the page parameter will tell us what page we are on, if no page is specified number one is assumed.
Let me show you how a request URL looks like and then break it down.
http://api.remix.bestbuy.com/v1/products(search=YOUR_QUERY)?apiKey=YOUR_API_KEY
The base URL for the search api is this
http://api.remix.bestbuy.com/v1/products
The products section acts like a function and its parameter is the word "search=" followed by the name of or type of product you are looking for.
http://api.remix.bestbuy.com/v1/products(search=YOUR_QUERY)
After that goes your apikey and page parameters. Copy and past the following URL in your browser, don’t forget to replace your API Key in it.
http://api.remix.bestbuy.com/v1/products(search=computers)?apiKey=YOUR_API_KEY
How To Parse The Result
If the previous step went right you should have gotten a response like this one in your browser. All this is XML now we can read it with PHP and display the results.
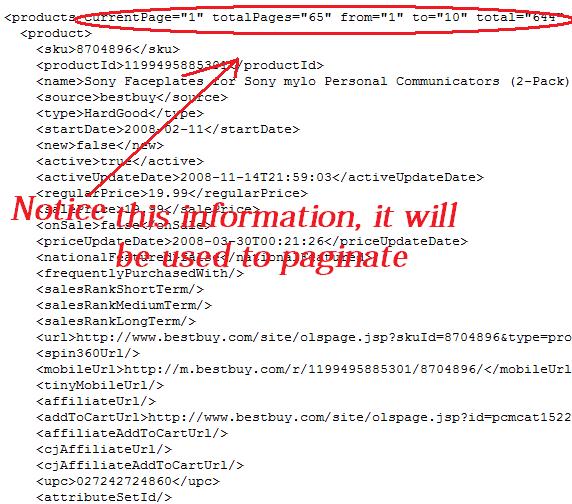
Best Buy API XML Response
I want to display each product’s name linked to their best buy pages, along with the price and thumbnail image. I also want a search box for people to query the API.
My form is pretty simple
<form action="" method="get"> <input type="text" name="query" /> <button type="submit">Search</button> </form>
This is how I will get the parameters I need.
$apiKey='YOUR_API_KEY'; $query=$_GET['query']; // user query
My request URL will have two forms, with and without a page parameter, this will depend on whether the parameter is set or not.
if(isset($_GET['page'])) { $currentPage=$_GET['page']; // need page paramter $requestUrl="http://api.remix.bestbuy.com/v1/products(search={$query})?apiKey={$apiKey}&page={$currentPage}"; } else { // without page parameter, 1st page $requestUrl="http://api.remix.bestbuy.com/v1/products(search={$query})?apiKey={$apiKey}"; }
Now I can retrieve the results and store them in an appropriately named variable. I will also get all the pagination information I will use.
$xml=simplexml_load_file($requestUrl); // get results $currentPage=$xml['currentPage']; $totalPages=$xml['totalPages']; $results=$xml['total'];
If you can’t figure out the code above and below check out my post on PHP and XML.
Let’s loop through the results with a foreach loop.
foreach($xml->product as $product) { echo " <fieldset> <p> <img src=\"{$product->thumbnailImage}\" alt=\"{$product->name}\"/> </p> Name: <a href=\"{$product->url}\">{$product->name}</a><br /> Sale Price: {$product->salePrice}<br /> Regular Price: {$product->salePrice}<br /> </fieldset>"; }
And now to get my pagination
for($i=1;$i<=$totalPages;$i++) { if($i!=$currentPage){ echo " <a href=\"?query={$query}&page={$i}\">{$i}</a> "; } else { echo " {$i} "; } }
The Full Script
I know that was REALLY hard to follow, I just wanted to show you each part of the script first. You have this script I made and do what you want with it. All you need to do is use your API key.
<?php $apiKey='xxxxxxxxxxxxxx'; if(isset($_GET['query'])) { $query=$_GET['query']; } else { echo ' <form action="" method="get"> <input type="text" name="query" /> <button type="submit">Search</button> </form> '; exit (0); } if(isset($_GET['page'])) { $currentPage=$_GET['page']; $requestUrl="http://api.remix.bestbuy.com/v1/products(search={$query})?apiKey={$apiKey}&page={$currentPage}"; } else { $requestUrl="http://api.remix.bestbuy.com/v1/products(search={$query})?apiKey={$apiKey}"; } echo $requestUrl; $xml=simplexml_load_file($requestUrl); $currentPage=$xml['currentPage']; $totalPages=$xml['totalPages']; $results=$xml['total']; ?> <html> <body> <form action="" method="get"> <input type="text" name="query" /> <button type="submit">Search</button> </form> <?php if($results>0) { foreach($xml->product as $product) { echo " <fieldset> <p> <img src=\"{$product->thumbnailImage}\" alt=\"{$product->name}\"/> </p> Name: <a href=\"{$product->url}\">{$product->name}</a><br /> Sale Price: {$product->salePrice}<br /> Regular Price: {$product->salePrice}<br /> </fieldset>"; } // pagination links for($i=1;$i<=$totalPages;$i++) { if($i!=$currentPage){ echo " <a href=\"?query={$query}&page={$i}\">{$i}</a> "; } else { echo " {$i} "; } } } else { echo 'Sorry, no products matched your criteria'; } ?> </body> </html>
The Result: A Best Buy Search Engine
If you got this point you will have the privilege to own a pretty neat best buy search engine.
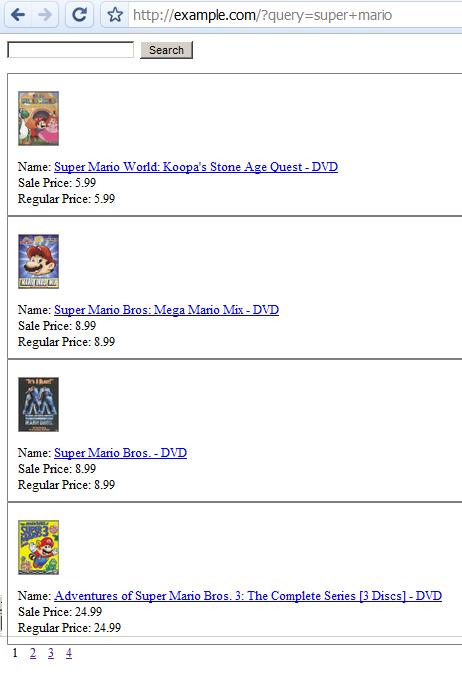
Your Own Best Buy Search Engine
The image only shows 4 results, that’s because I had to delete some so that I could fit the pagination links in the image.